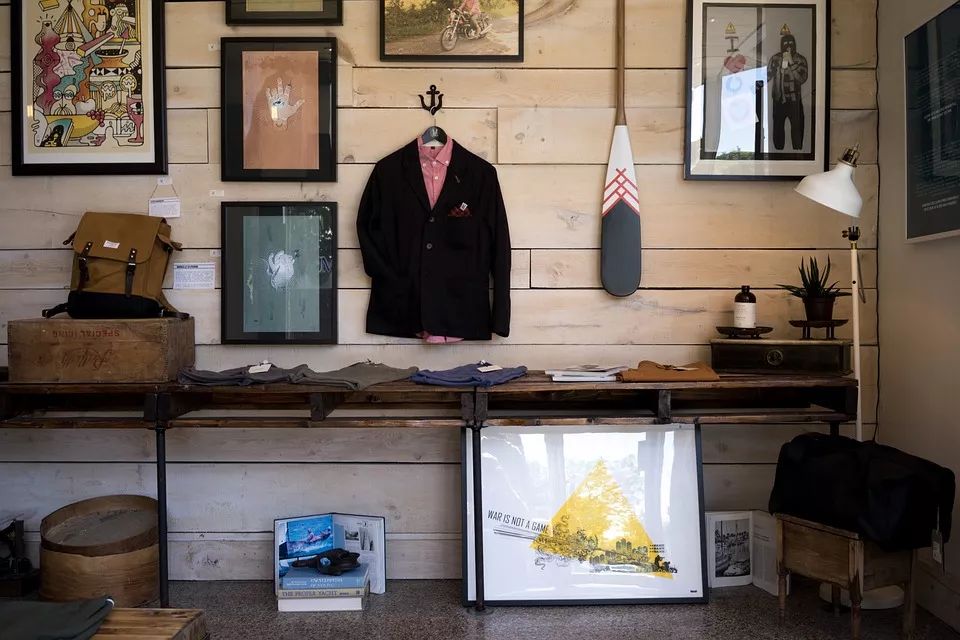
文 | Brendan Maginnis 译 | EarlGrey
推荐 | 编程派公众号(ID:codingpy)
原文:https://sourcery.ai/blog/python-best-practices/
在开始一个新的Python项目时,很容易不做规划直接进入编码环节。花费少量时间,用最好的工具设置项目,将节省大量时间并带来更快乐的编码体验。
在理想的世界中,所有开发人员使用的依赖库都是相同的,代码将被完美地格式化,禁止常见错误,并且测试将涵盖所有内容。此外,每次提交代码时都会确保符合这些要求。
在本文中,我将介绍如何设置一个这样的理想项目。你可以跟随我的步骤操作,也可以直接开始安装pipx和pipenv,然后生成新项目。
让我们创建一个新的项目目录:
mkdir best_practices
cd best_practices
Python命令行工具与pipx
Pipx是一个方便的实用程序,允许快速安装python命令行工具。我们将用它来安装 pipenv 和 cookiecutter 。
python3 -m pip install --user pipx
python3 -m pipx ensurepath
使用 pipenv 进行依赖管理
Pipenv自动为您的项目创建和管理virtualenv,以及在安装/卸载软件包时从Pipfile添加/删除软件包。它还生成了非常重要的Pipfile.lock文件,用于生成确定性构建。
知道你和你的同事正在使用相同的库版本,可以极大提升信心。Pipenv 可以实现这个点,因此在过去一年多里得到了大量开发者的青睐
pipx install pipenv
使用 black 和 isort 进行代码格式化
Black是代码格式化工具:
Black是不妥协的Python代码格式化程序。通过使用它,意味着您同意放弃对手动格式化细节的控制。作为回报,Black 为你提供速度和确定性,并且无需处理 pycodestyle 的繁琐提示。你将有更多的时间,来处理更重要的事情。
无论是什么项目,Black 格式化后的代码看起来都是一样的。习惯之后,你不会再注意到格式的问题,可以专注于内容。
Black产生的代码差异最小,可以加速代码审查。
isort则用来处理 import 的排序:
isort是可以按字母顺序对 import 进行排序,并自动分成多个部分。
让我们使用pipenv安装它们为开发依赖库,这样就不会让部署版本变得更复杂:
pipenv install black isort --dev
Black 和 isort 的默认选项之间有冲突,因此我们将覆盖 isort 的选项配置,使用 Black 的配置。创建一个 setup.cfg
文件并添加此配置:
[isort]
multi_line_output=3
include_trailing_comma=True
force_grid_wrap=0
use_parentheses=True
line_length=88
我们可以这样运行这些工具:
pipenv run black
pipenv run isort
用flake8强化风格
Flake8确保我们的代码遵循PEP8的约定。使用pipenv安装:
pipenv install flake8 --dev
就像isort一样,它需要一些配置才能与 Black 配合使用。将此配置添加到 setup.cfg
:
[flake8]
ignore = E203,E266,E501,W503
max-line-length = 88
max-complexity = 18
select = B,C,E,F,W,T4
现在我们可以使用 pipenv run flake8
运行flake8。
使用 mypy 检查静态类型
Mypy是Python的一个可选静态类型检查器,旨在结合动态(或“鸭子”)类型和静态类型的好处。Mypy将Python的表现力和便利性与强大的类型系统和编译时类型检查相结合。Mypy对标准Python程序进行类型检查,使用 Python VM 运行 mypy 基本没有运行时的开销。
在Python中使用类型需要慢慢习惯,但好处是巨大的。mypy 官网这样写道:
pipenv install mypy --dev
默认情况下,Mypy将递归检查所有类型注释的导入,这会导致库不包含这些注释时出错。我们需要将mypy配置为仅在我们的代码上运行,并忽略没有类型注释的导入的任何错误。我们假设代码存在于以下配置的 best_practices
包中。将如下配置添加到 setup.cfg
:
[mypy]
files=best_practices,test
ignore_missing_imports=true
现在我们可以运行mypy:
pipenv run mypy
mypy 的速查表:https://mypy.readthedocs.io/en/latest/cheatsheetpy3.html
使用pytest和pytest-cov进行测试
使用pytest编写测试非常容易,并且消除编写测试的阻力,意味着我们会编写更多的测试!
pipenv install pytest pytest-cov --dev
以下是pytest网站的一个简单示例:
# content of test_sample.py
def inc(x):
return x + 1
def test_answer():
assert inc(3) == 5
执行示例:
$ pipenv run pytest
=========================== test session starts ============================
platform linux -- Python
3.x.y, pytest-5.x.y, py-1.x.y, pluggy-0.x.y
cachedir: $PYTHON_PREFIX/.pytest_cache
rootdir: $REGENDOC_TMPDIR
collected 1 item
test_sample.py F [100%]
================================= FAILURES =================================
_______________________________ test_answer ________________________________
def test_answer():
> assert inc(3) == 5
E assert 4 == 5
E + where 4 = inc(3)
test_sample.py:6: AssertionError
========================= 1 failed in 0.12 seconds =========================
所有的测试都应该放在 test
目录中,所以将这个配置添加到 setup.cfg
:
[tool:pytest]
testpaths=test
我们还想检查测试覆盖了多少代码。创建一个新文件 .coveragerc
,用来返回应用程序代码的覆盖率统计信息,我们再次假设代码位于 best_practices
模块中:
[run]
source = best_practices
[report]
exclude_lines =
# Have to re-enable the standard pragma
pragma: no cover
# Don't complain about missing debug-only code:
def __repr__
if self\.debug
# Don't complain if tests don't hit defensive assertion code:
raise AssertionError
raise NotImplementedError
# Don't complain if non-runnable code isn't run:
if 0:
if __name__ == .__main__.:
我们现在可以运行测试并报告覆盖率
pipenv run pytest --cov --cov-fail-under=100
如果对应用程序代码的测试覆盖率低于100%,则会失败。
pre-commit 的 Git 钩子
Git钩子允许您在任何时候提交或推送时运行脚本。这就可以支持我们在每次提交/推送时,自动运行所有的格式化和测试。pre-commit可以帮助我们轻松配置这些钩子:
在提交代码审查之前,Git钩子脚本可以帮助识别简单问题。每次提交时运行钩子,自动指出代码中的问题,例如缺少分号,尾随空格和调试语句。在代码审查之前指出这些问题,可以让代码审查者专注于代码架构的变化,而不是浪费时间检查格式问题。
在这里,我们配置在提交Python 文件修改时,执行上述所有检查,并且仅在推送时运行pytest覆盖率测试,因为耗时可能较长。创建一个新文件 .pre-commit-config.yaml
:
repos:
- repo: local
hooks:
- id: isort
name: isort
stages: [commit]
language: system
entry: pipenv run isort
types: [python]
- id: black
name: black
stages: [commit]
language: system
entry: pipenv run black
types: [python]
- id: flake8
name: flake8
stages: [commit]
language: system
entry: pipenv run flake8
types: [python]
exclude: setup.py
- id: mypy
name: mypy
stages: [commit]
language: system
entry: pipenv run mypy
types: [python]
pass_filenames: false
- id: pytest
name: pytest
stages: [commit]
language: system
entry: pipenv run pytest
types: [python]
- id: pytest-cov
name: pytest
stages: [push]
language: system
entry: pipenv run pytest --cov --cov-fail-under=100
types: [python]
pass_filenames: false
如果你需要跳过这些钩子,你可以运行 git commit--no-verify
或 git push--no-verify
使用cookiecutter生成项目
我们已经看到了理想项目都使用了哪些工具,可以将其固化为一个模板,只需要1个命令 即可生成新项目:
pipx run cookiecutter gh:sourceryai/python-best-practices-cookiecutter
填写项目名称和仓库名称,即可使用模板为你生成项目。
要完成设置,请按照下列步骤操作:
# Enter project directory
cd
# Initialise git repo
git init
# Install dependencies
pipenv install --dev
# Setup pre-commit and pre-push hooks
pipenv run pre-commit install -t pre-commit
pipenv run pre-commit install -t pre-push
模板项目包含一个非常简单的Python文件和测试来试用这些工具。一旦你对代码感到满意,你就可以做第一个 git commit
,这时所有的钩子都会运行。
回复下方「关键词」,获取优质资源
回复关键词「 pybook03」,立即获取主页君与小伙伴一起翻译的《Think Python 2e》电子版
回复关键词「pybooks02」,立即获取 O'Reilly 出版社推出的免费 Python 相关电子书合集
回复关键词「书单02」,立即获取主页君整理的 10 本 Python 入门书的电子版

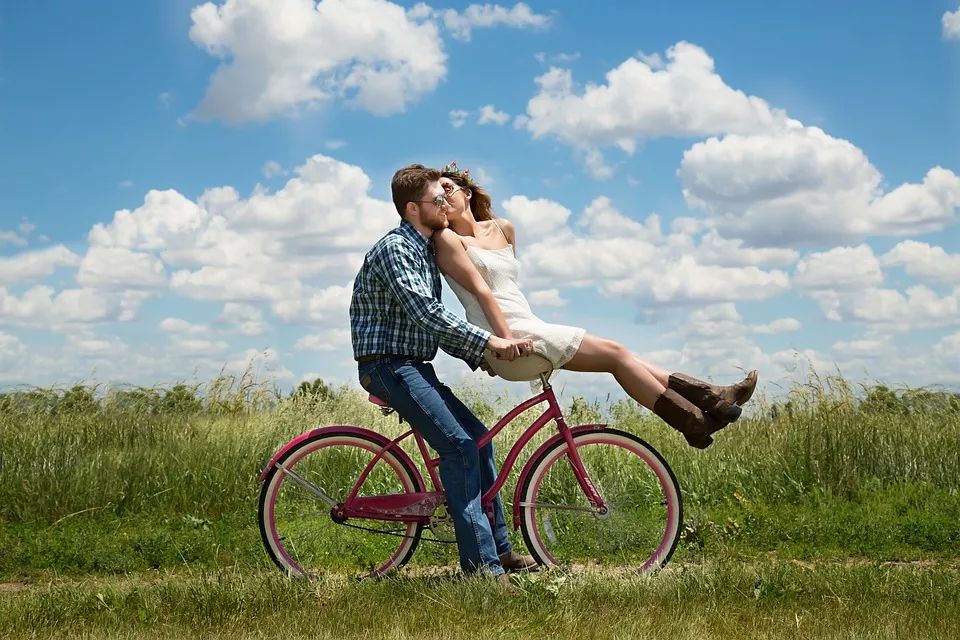
豆瓣 9.1 分,中文版销量 30 多万,零基础也能用这本书学会 Python
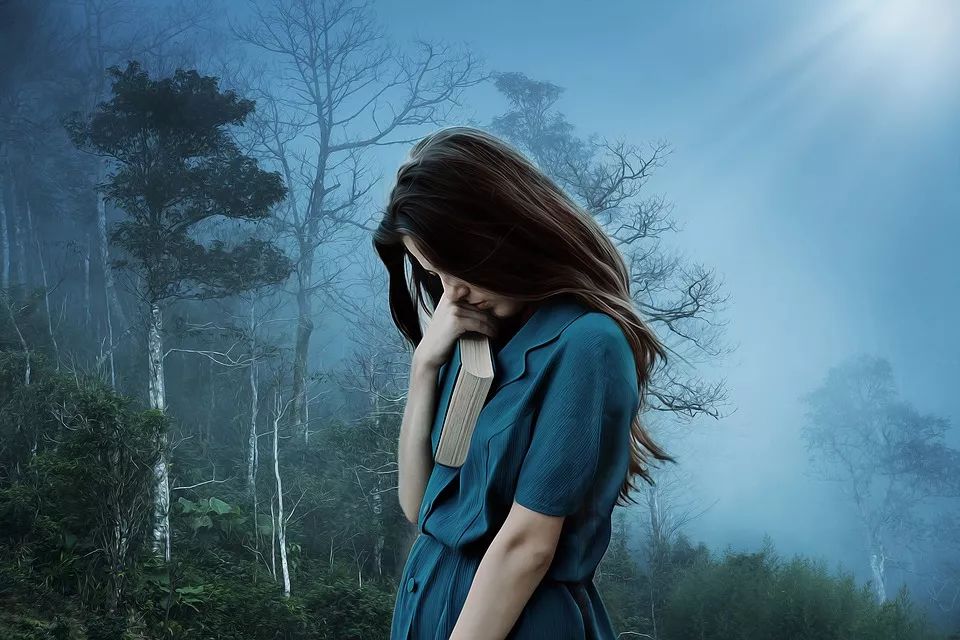
你想要的 IT 电子资源,这里可能都有
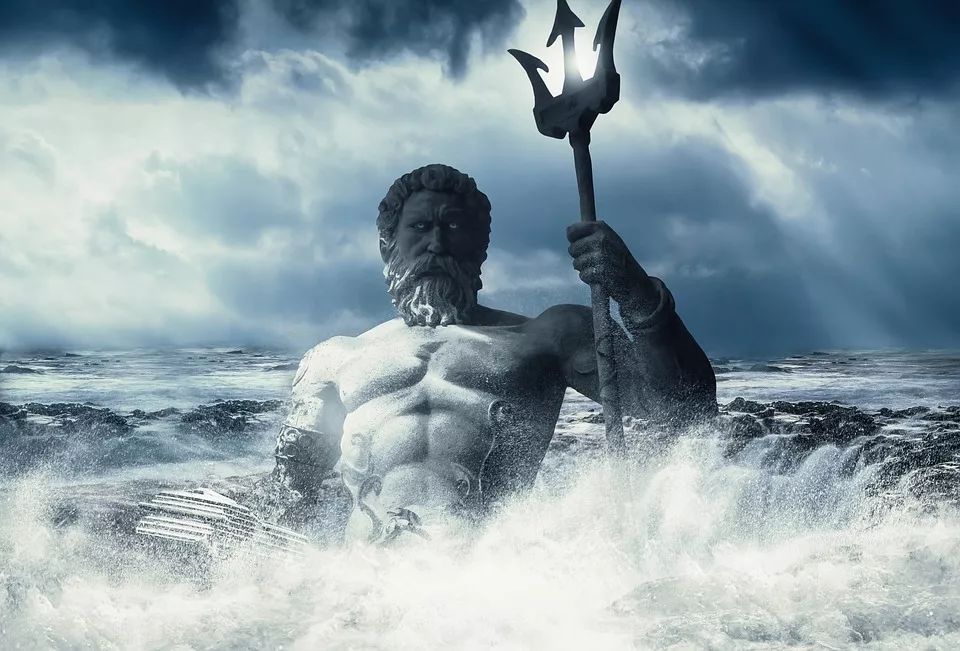
Python 或将超越 C、Java,成为最受欢迎的语言
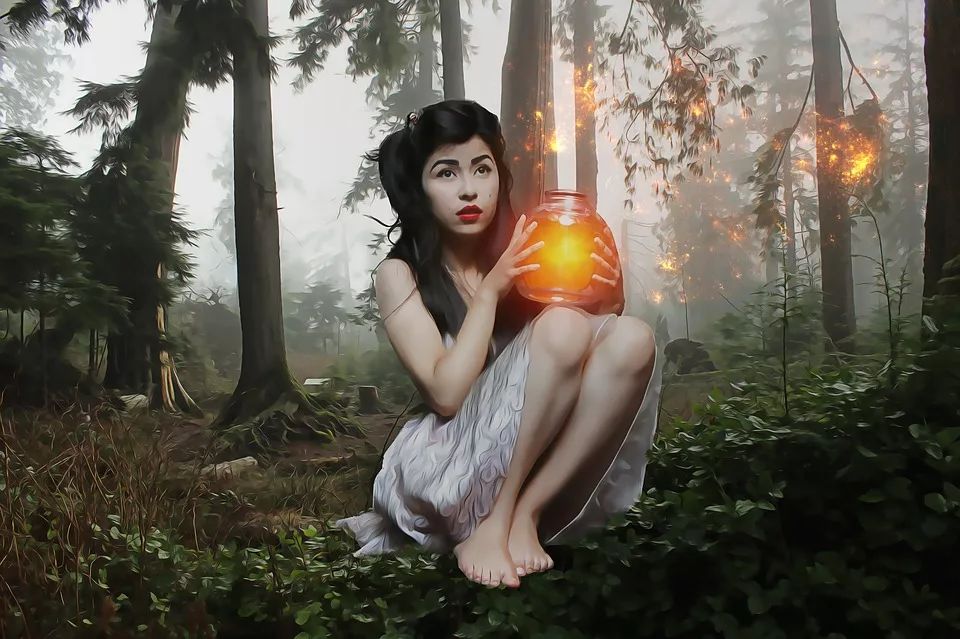
Python 容器使用的 5 个技巧和 2 个误区
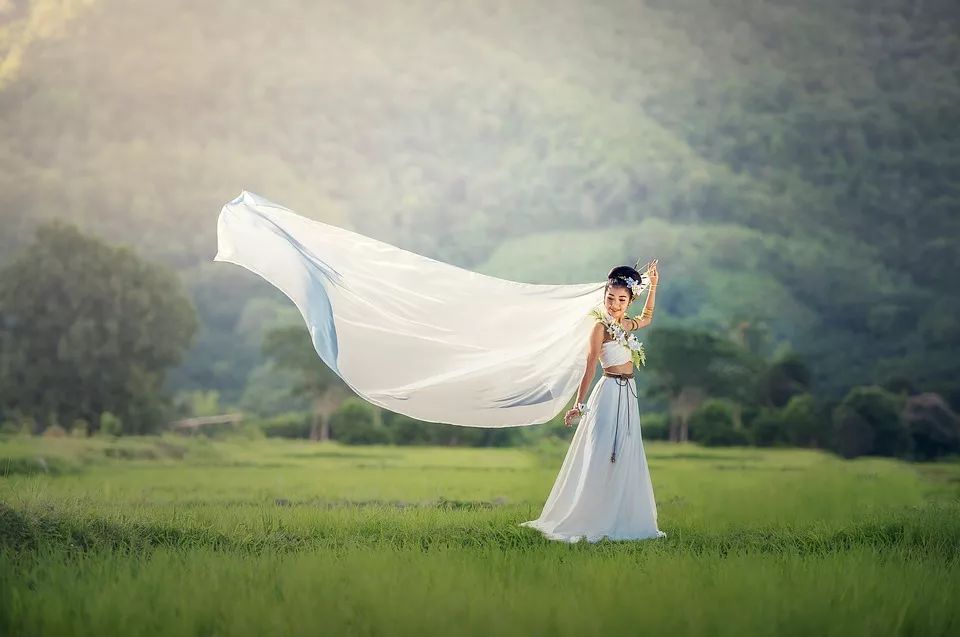
如何写出优雅的 Python 函数?
题图:pexels,CC0 授权。
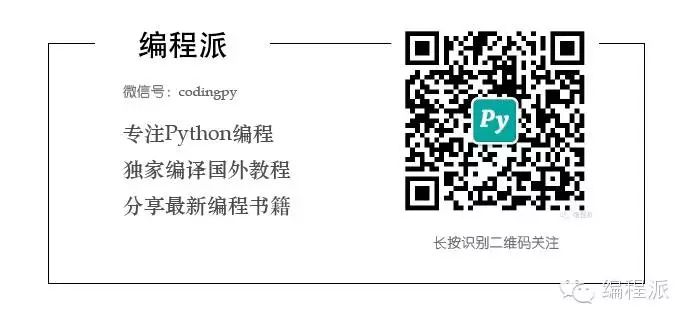