动态分类器选择是一种用于分类预测建模的集成学习算法。该技术涉及在训练数据集上拟合多个机器学习模型,然后基于要预测的示例的特定细节,选择在进行预测时预期表现最佳的模型。这可以通过以下方法实现:使用k最近邻模型在训练数据集中找到最接近要预测的新示例的示例,评估该邻域中池中的所有模型,并使用在邻域中表现最佳的模型来对新示例做出预测。这样,动态分类器选择通常可以比池中的任何单个模型更好地执行,并且提供了对来自多个模型的预测求平均的另一种方法,就像其他集成算法一样。在本教程中,您将发现如何在Python中开发动态分类器选择集合。动态分类器选择算法从众多模型中选择一种以对每个新示例进行预测。
如何使用scikit-learn API开发和评估用于分类任务的动态分类器选择模型。
如何探索动态分类器选择模型超参数对分类准确性的影响。
动态分类器选择
使用Scikit-Learn进行动态分类器选择
具有整体本地精度(OLA)的DCS
具有本地分类精度(LCA)的DCS
DCS的超参数调整
在k最近邻居中探索k
探索分类器池的算法
多个分类器系统是指机器学习算法的一个领域,该算法使用多个模型来解决分类预测建模问题。这包括熟悉的技术,如“一对多休息”,“一对多所有”和输出纠错码技术。它还包括更通用的技术,这些技术选择模型以动态地用于需要预测的每个新示例。Dynamic Ensemble Selection Library
或简称为DESlib
是一个开放源代码Python库(https://github.com/scikit-learn-contrib/DESlib
),它提供了许多不同的动态分类器选择算法的实现。DESlib是一个易于使用的集成学习库,致力于实现动态分类器和集成选择的最新技术。pip install deslib
安装完成后,我们可以通过加载库并打印已安装的版本来确认该库已正确安装并可以使用。验证截图如下所示表示可以正常使用了: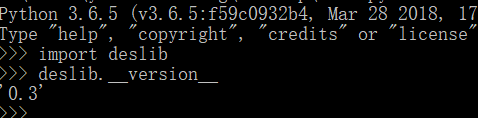
DESlib
通过每种分类器选择技术分别通过OLA和LCA类提供了DCS-LA
算法的实现。每个类都可以直接用作scikit学习模型,从而可以直接使用全套scikit学习数据准备,建模管道和模型评估技术。这两个类都使用k最近邻居算法来选择默认值k = 7
的邻居。尽管可以通过将“ pool_classifiers”
设置为模型列表来更改,但决策树的自举聚合(装袋)合奏用作针对每个分类考虑的分类器模型池。我们可以使用make_classification()
函数创建具有10,000个示例和20个输入功能的综合二进制分类问题。其中,OLA和LCA解释如下:
局部精度,通常称为LA或整体本地精度(OLA)。
类精度,通常称为CA或本地类精度(LCA)。
局部精度(OLA)涉及评估k个训练示例附近的每个模型的分类精度。 然后选择在该邻域中表现最佳的模型以对新示例进行预测。
类精度(LCA)涉及使用每种模型对新示例进行预测,并记录所预测的类。 然后,评估每个模型在k个训练示例的邻居上的准确性,并选择对新示例所预测的类具有最佳技能的模型,并返回其预测。
# synthetic binary classification dataset
from sklearn.datasets import make_classification
# define dataset
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# summarize the dataset
print(X.shape, y.shape)
(10000, 20) (10000,)
我们可以使用综合数据集上的整体局部精度来评估DCS-LA
模型。在这种情况下,我们将使用默认的模型超参数,包括袋装决策树作为分类器模型的池,并且在进行预测时将k = 7用于选择局部邻域。我们将使用具有三个重复和10折的重复分层k折交叉验证来评估模型。我们将报告所有重复和折叠中模型准确性的均值和标准差。# evaluate dynamic classifier selection DCS-LA with overall local accuracy
from numpy import mean
from numpy import std
from sklearn.datasets import make_classification
from sklearn.model_selection import cross_val_score
from sklearn.model_selection import RepeatedStratifiedKFold
from deslib.dcs.ola import OLA
# define dataset
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# define the model
model = OLA()
# define the evaluation procedure
cv = RepeatedStratifiedKFold(n_splits=10, n_repeats=3, random_state=1)
# evaluate the model
n_scores = cross_val_score(model, X, y, scoring='accuracy', cv=cv, n_jobs=-1)
# report performance
print('Mean Accuracy: %.3f (%.3f)' % (mean(n_scores), std(n_scores)))
注意:由于算法或评估程序的随机性,或者数值精度的差异,您的结果可能会有所不同。考虑运行该示例几次并比较平均结果。在这种情况下,我们可以看到带有OLA和默认超参数的DCS-LA达到了约88.3%的分类精度。Mean Accuracy: 0.883 (0.012)
我们还可以将DCS-LA模型与OLA一起用作最终模型,并进行分类预测。首先,模型适合所有可用数据,然后可以调用predict()
函数对新数据进行预测。下面的示例在我们的二进制分类数据集中展示了这一点。# make a prediction with DCS-LA using overall local accuracy
from sklearn.datasets import make_classification
from deslib.dcs.ola import OLA
# define dataset
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# define the model
model = OLA()
# fit the model on the whole dataset
model.fit(X, y)
# make a single prediction
row = [0.2929949,-4.21223056,-1.288332,-2.17849815,-0.64527665,2.58097719,0.28422388,-7.1827928,-1.91211104,2.73729512,0.81395695,3.96973717,-2.66939799,3.34692332,4.19791821,0.99990998,-0.30201875,-4.43170633,-2.82646737,0.44916808]
yhat = model.predict([row])
print('Predicted Class: %d' % yhat[0])
运行示例使DCS-LA和OLA模型适合整个数据集,然后用于对新的数据行进行预测,就像在应用程序中使用模型时一样。我们可以在合成数据集上使用局部类的准确性评估DCS-LA模型。在这种情况下,我们将使用默认的模型超参数,包括袋装决策树作为分类器模型的池,并且在进行预测时将k = 7
用于选择局部邻域。我们将使用具有三个重复和10折的重复分层k折交叉验证来评估模型。我们将报告所有重复和折叠中模型准确性的均值和标准差。
# evaluate dynamic classifier selection DCS-LA using local class accuracy
from numpy import mean
from numpy import std
from sklearn.datasets import make_classification
from sklearn.model_selection import cross_val_score
from sklearn.model_selection import RepeatedStratifiedKFold
from deslib.dcs.lca import LCA
# define dataset
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# define the model
model = LCA()
# define the evaluation procedure
cv = RepeatedStratifiedKFold(n_splits=10, n_repeats=3, random_state=1)
# evaluate the model
n_scores = cross_val_score(model, X, y, scoring='accuracy', cv=cv, n_jobs=-1)
# report performance
print('Mean Accuracy: %.3f (%.3f)' % (mean(n_scores), std(n_scores)))
注意:由于算法或评估程序的随机性,或者数值精度的差异,您的结果可能会有所不同。考虑运行该示例几次并比较平均结果。在这种情况下,我们可以看到带有LCA和默认超参数的DCS-LA达到了约92.2%的分类精度。看到带有LCA和默认超参数的DCS-LA达到了约92.2%的分类精度。
我们还可以将带有LCA的DCS-LA模型用作最终模型,并进行分类预测。首先,模型适合所有可用数据,然后可以调用predict()
函数对新数据进行预测。下面的示例在我们的二进制分类数据集中展示了这一点。# make a prediction with DCS-LA using local class accuracy
from sklearn.datasets import make_classification
from deslib.dcs.lca import LCA
# define dataset
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# define the model
model = LCA()
# fit the model on the whole dataset
model.fit(X, y)
# make a single prediction
row = [0.2929949,-4.21223056,-1.288332,-2.17849815,-0.64527665,2.58097719,0.28422388,-7.1827928,-1.91211104,2.73729512,0.81395695,3.96973717,-2.66939799,3.34692332,4.19791821,0.99990998,-0.30201875,-4.43170633,-2.82646737,0.44916808]
yhat = model.predict([row])
print('Predicted Class: %d' % yhat[0])
运行示例可以使DCS-LA和LCA模型适合整个数据集,然后用于对新数据行进行预测,就像我们在应用程序中使用模型时一样。在本节中,我们将仔细研究一些您应该考虑调整DCS-LA模型的超参数及其对模型性能的影响。对于DCS-LA,我们可以查看许多超参数,尽管在这种情况下,我们将查看模型局部评估中使用的k最近邻居模型中的k值,以及如何使用自定义的分类器。我们将使用带有OLA的DCS-LA作为这些实验的基础,尽管具体方法的选择是任意的。k近邻算法的配置对DCS-LA模型至关重要,因为它定义了考虑每个分类器进行选择的邻域范围。k值控制邻域的大小,重要的是将其设置为适合您的数据集的值,特别是特征空间中样本的密度。值太小将意味着可能会将训练集中的相关示例从邻域中排除,而值太大可能意味着信号被太多示例所冲走。以下示例探讨了kS值为2到21的带OLA的DCS-LA的分类精度。# explore k in knn for DCS-LA with overall local accuracy
from numpy import mean
from numpy import std
from sklearn.datasets import make_classification
from sklearn.model_selection import cross_val_score
from sklearn.model_selection import RepeatedStratifiedKFold
from deslib.dcs.ola import OLA
from matplotlib import pyplot
# get the dataset
def get_dataset():
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
return X, y
# get a list of models to evaluate
def get_models():
models = dict()
for n in range(2,22):
models[str(n)] = OLA(k=n)
return models
# evaluate a give model using cross-validation
def evaluate_model(model):
cv = RepeatedStratifiedKFold(n_splits=10, n_repeats=3, random_state=1)
scores = cross_val_score(model, X, y, scoring='accuracy', cv=cv, n_jobs=-1)
return scores
# define dataset
X, y = get_dataset()
# get the models to evaluate
models = get_models()
# evaluate the models and store results
results, names = list(), list()
for name, model in models.items():
scores = evaluate_model(model)
results.append(scores)
names.append(name)
print('>%s %.3f (%.3f)' % (name, mean(scores), std(scores)))
# plot model performance for comparison
pyplot.boxplot(results, labels=names, showmeans=True)
pyplot.show()
首先运行示例将报告每个配置的邻域大小的平均准确性。注意:由于算法或评估程序的随机性,或者数值精度的差异,您的结果可能会有所不同。考虑运行该示例几次并比较平均结果。在这种情况下,我们可以看到,精度随着邻域大小的增加而增加,可能达到k = 13或k = 14,在此看来它趋于平稳>2 0.873 (0.009)
>3 0.874 (0.013)
>4 0.880 (0.009)
>5 0.881 (0.009)
>6 0.883 (0.010)
>7 0.883 (0.011)
>8 0.884 (0.012)
>9 0.883 (0.010)
>10 0.886 (0.012)
>11 0.886 (0.011)
>12 0.885 (0.010)
>13 0.888 (0.010)
>14 0.886 (0.009)
>15 0.889 (0.010)
>16 0.885 (0.012)
>17 0.888 (0.009)
>18 0.886 (0.010)
>19 0.889 (0.012)
>20 0.889 (0.011)
>21 0.886 (0.011)
创建箱须图以分配每个配置的邻域大小的准确性得分。我们可以看到在达到平稳之前,模型性能和k值增加的总体趋势选择DCS-LA池中使用的算法是另一个重要的超参数。默认情况下,使用袋装决策树,因为事实证明它是处理一系列分类任务的有效方法。不过,可以考虑使用自定义分类器池。这需要首先定义分类器模型的列表,以使用每个分类器模型并将其拟合到训练数据集上。不幸的是,这意味着在这种情况下不能使用scikit-learn中的自动k折交叉验证模型评估方法。取而代之的是,我们将使用训练测试拆分,以便我们可以在训练数据集上手动拟合分类器池。然后,可以通过“ pool_classifiers”
参数将适合分类器的列表指定给OLA(或LCA)类。在这种情况下,我们将使用一个包含逻辑回归,决策树和朴素贝叶斯分类器的池。下面列出了使用OLA和综合数据集中的一组自定义分类器评估DCS-LA的完整示例。# evaluate DCS-LA using OLA with a custom pool of algorithms
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from deslib.dcs.ola import OLA
from sklearn.linear_model import LogisticRegression
from sklearn.tree import DecisionTreeClassifier
from sklearn.naive_bayes import GaussianNB
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# split the dataset into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.5, random_state=1)
# define classifiers to use in the pool
classifiers = [
LogisticRegression(),
DecisionTreeClassifier(),
GaussianNB()]
# fit each classifier on the training set
for c in classifiers:
c.fit(X_train, y_train)
# define the DCS-LA model
model = OLA(pool_classifiers=classifiers)
# fit the model
model.fit(X_train, y_train)
# make predictions on the test set
yhat = model.predict(X_test)
# evaluate predictions
score = accuracy_score(y_test, yhat)
print('Accuracy: %.3f' % (score))
首先运行示例将使用自定义分类器报告模型的平均准确性。注意:由于算法或评估程序的随机性,或者数值精度的差异,您的结果可能会有所不同。考虑运行该示例几次并比较平均结果。在这种情况下,我们可以看到该模型的准确度约为91.2%。
为了采用DCS模型,其性能必须优于任何贡献模型。否则,我们将仅使用性能更好的贡献模型。我们可以通过评估测试集中每个贡献分类器的性能来检查这一点。...
# evaluate contributing models
for c in classifiers:
yhat = c.predict(X_test)
score = accuracy_score(y_test, yhat)
print('>%s: %.3f' % (c.__class__.__name__, score))
下面列出了带有自定义分类器池的DCS-LA的更新示例,该分类器也进行了独立评估。# evaluate DCS-LA using OLA with a custom pool of algorithms
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from deslib.dcs.ola import OLA
from sklearn.linear_model import LogisticRegression
from sklearn.tree import DecisionTreeClassifier
from sklearn.naive_bayes import GaussianNB
# define dataset
X, y = make_classification(n_samples=10000, n_features=20, n_informative=15, n_redundant=5, random_state=7)
# split the dataset into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.5, random_state=1)
# define classifiers to use in the pool
classifiers = [
LogisticRegression(),
DecisionTreeClassifier(),
GaussianNB()]
# fit each classifier on the training set
for c in classifiers:
c.fit(X_train, y_train)
# define the DCS-LA model
model = OLA(pool_classifiers=classifiers)
# fit the model
model.fit(X_train, y_train)
# make predictions on the test set
yhat = model.predict(X_test)
# evaluate predictions
score = accuracy_score(y_test, yhat)
print('Accuracy: %.3f' % (score))
# evaluate contributing models
for c in classifiers:
yhat = c.predict(X_test)
score = accuracy_score(y_test, yhat)
print('>%s: %.3f' % (c.__class__.__name__, score))
首先运行示例,报告使用定制分类器池的模型的平均准确性以及每个贡献模型的准确性。注意:由于算法或评估程序的随机性,或者数值精度的差异,您的结果可能会有所不同。考虑运行该示例几次并比较平均结果。在这种情况下,我们可以再次看到,DCS-LA的精度达到了约91.3%,比任何贡献模型都要好。Accuracy: 0.913
>LogisticRegression: 0.878
>DecisionTreeClassifier: 0.884
>GaussianNB: 0.873