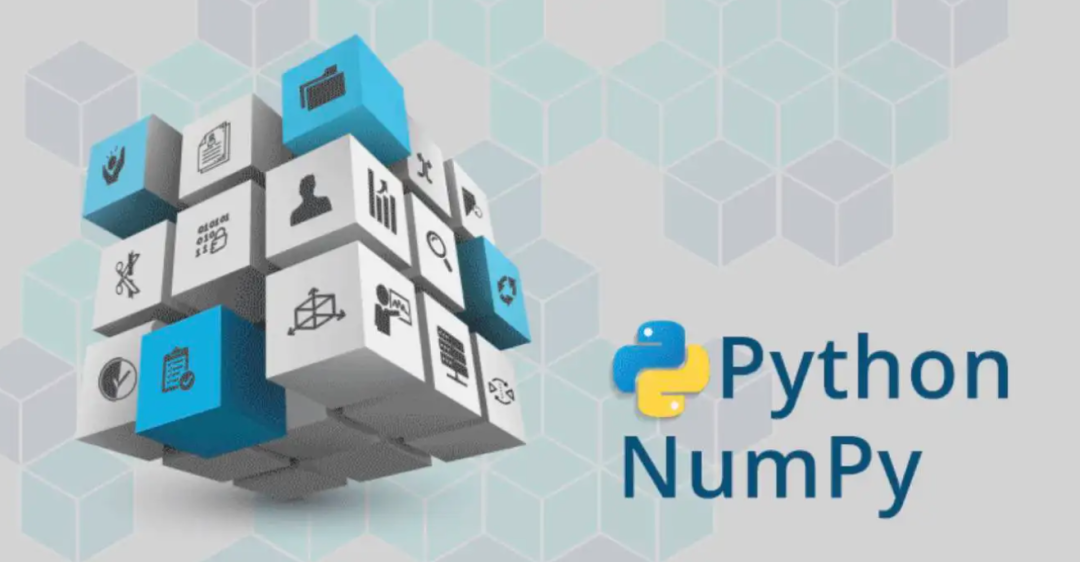
ndarray的基本操作
1. 索引
一维与列表完全一致, 多维度同理
# 列表
l = [1,2,3,4,5,6]
l[3]
# 输出
# 4
# numpy数组(ndarray类型)
n = np.array(l)
n[3]
# 输出
# 4
# 二维数组
n = np.random.randint(0,10, size=(4,5))
n
# array([[1, 2, 5, 1, 5],
# [5, 5, 6, 9, 8],
# [3, 4, 2, 2, 0],
# [4, 4, 8, 4, 3]])
# 找到3
n[3][4]
n[-1][-1]
# 简写
n[3,4]
n[-1,-1]
# 三维数组
n = np.random.randint(0, 100, size=(4,5,6))
# 3个维度
n[2,2,3]
n[-2,-3,3]
根据索引修改数据
# 定位到指定元素,直接修改
n[2,2,3] = 6666
# 修改一个数组
n[0,0] = [1, 2, 3]
n = np.zeros((6,6), dtype=int)
n
# 输出
# array([[0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0]])
# 修改1行
n[0] = 1
n
# 输出
# array([[1, 1, 1, 1, 1, 1],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0]])
# 修改多行
n[[0,3,-1]] = 2
n
# 输出
# array([[2, 2, 2, 2, 2, 2],
# [0, 0, 0, 0, 0, 0],
# [0, 0, 0, 0, 0, 0],
# [2, 2, 2, 2, 2, 2],
# [0, 0, 0, 0, 0, 0],
# [2, 2, 2, 2, 2, 2]])
2. 切片
一维与列表完全一致 多维度同理
# 列表切片
l = [1,2,3,4,5,6]
l[::-1]
# 输出
# [6, 5, 4, 3, 2, 1]
# 一维数组
n = np.arange(10)
n
# 输出
# array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
n[::2]
# 输出
# array([0, 2, 4, 6, 8])
n[::-1]
# 输出
# array([9, 8, 7, 6, 5, 4, 3, 2, 1, 0])
# 多维数组
n = np.random.randint(0,100, size=(5,6))
n
# 输出
# array([[94, 45, 60, 71, 70, 88],
# [43, 16, 39, 70, 14, 4],
# [59, 12, 84, 38, 96, 88],
# [80, 9, 72, 95, 69, 91],
# [44, 84, 5, 47, 92, 31]])
# 行 翻转
n[::-1]
# 列 翻转 (第二个维度)
n[:, ::-1]
实例: 把猫图片翻转
# cat.jpg
cat = plt.imread('cat.jpg')
#[[[231 186 131]
# [232 187 132]
# [233 188 133]
# ...
# [100 54 54]
# [ 92 48 47]
# [ 85 43 44]]
# ...
# ]
cat.shape
# 图片: 3维
# (456, 730, 3)
# 显示图片
plt.imshow(cat)
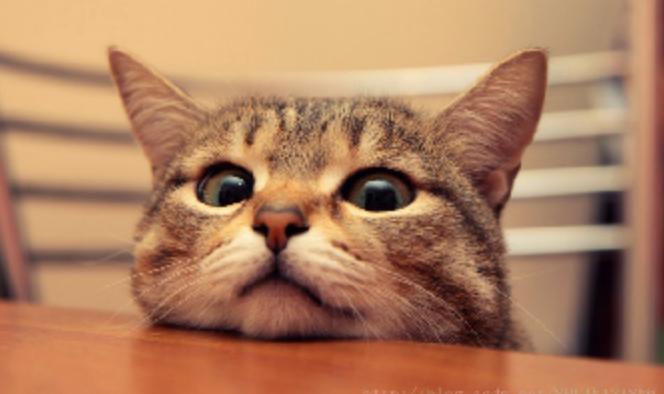
# 上下翻转
plt.imshow(cat[::-1])
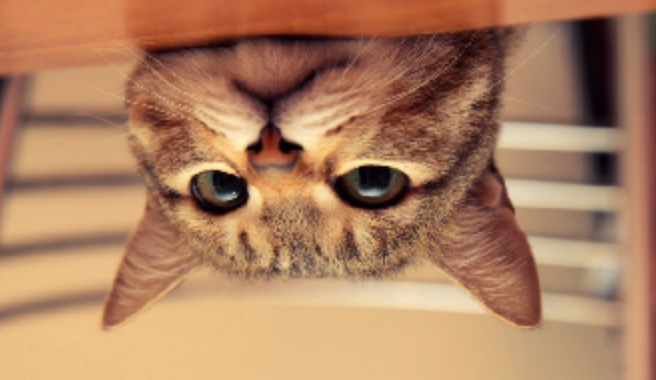
# 左右翻转
plt.imshow(cat[:,::-1])
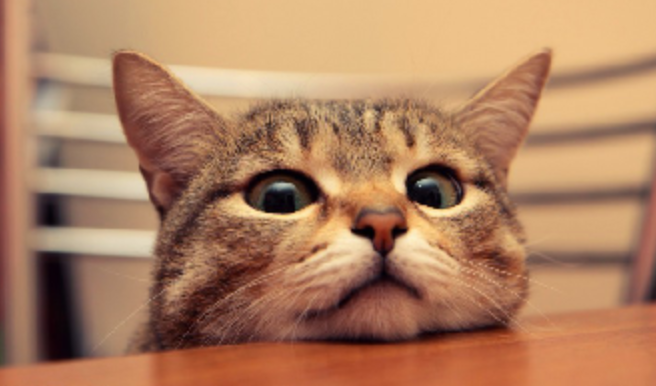
# 第三个维度翻转,颜色翻转,模糊处理
plt.imshow(cat[::10,::10,::-1])
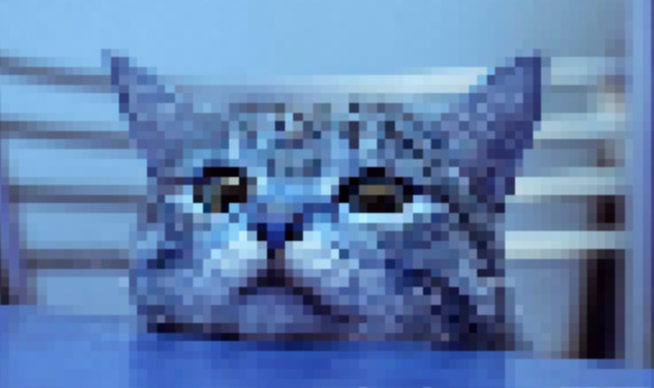
3. 变形
使用reshape函数, 注意参数是一个tuple
n = np.arange(1, 21)
n
# array([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20])
n.shape
# (20,)
# 变成2维
n2 = np.reshape(n, (4,5))
n2
# array([[ 1, 2, 3, 4, 5],
# [ 6, 7, 8, 9, 10],
# [11, 12, 13, 14, 15],
# [16, 17, 18, 19, 20]])
n2.shape
# (4, 5)
n2.reshape((20,)) # 变成一维
n2.reshape((-1,)) # 变成一维
# 改变cat的形状
cat.shape
# (456, 730, 3)
# -1 表示行会自动分配,列数是6
cat2 = cat.reshape((-1, 6))
cat2.shape
# (166440, 6)
# -1 表示列会自动分配,行数是6
cat2 = cat.reshape((6,-1))
cat2.shape
# (6, 166440)
4. 级联
concatenate
n1 = np.random.randint(0,100, size=(4,5))
n2 = np.random.randint(0,100, size=(4,5))
display(n1, n2)
# array([[48, 89, 82, 88, 55],
# [63, 80, 77, 27, 51],
# [11, 77, 90, 23, 71],
# [ 4, 11, 19, 84, 57]])
#
#array([[86, 26, 71, 62, 46],
# [75, 43, 84, 87, 99],
# [34, 33, 58, 56, 29],
# [56, 32, 53, 43, 5]])
# 级联,合并
# 上下合并:垂直级联
np.concatenate((n1, n2))
np.concatenate((n1, n2), axis=0) # axis=0表示行,第一个维度
# array([[48, 89, 82, 88, 55],
# [63, 80, 77, 27, 51],
# [11, 77, 90, 23, 71],
# [ 4, 11, 19, 84, 57],
# [86, 26, 71, 62, 46],
# [75, 43, 84, 87, 99],
# [34, 33, 58, 56, 29],
# [56, 32, 53, 43, 5]])
# 左右合并:水平级联
np.concatenate((n1, n2), axis=1) # axis=1表示列,第二个维度
# array([[48, 89, 82, 88, 55, 86, 26, 71, 62, 46],
# [63, 80, 77, 27, 51, 75, 43, 84, 87, 99],
# [11, 77, 90, 23, 71, 34, 33, 58, 56, 29],
# [ 4, 11, 19, 84, 57, 56, 32, 53, 43, 5]])
np.hstack与np.vstack
# 左右合并:水平级联
np.hstack((n1, n2))
# array([[48, 89, 82, 88, 55, 86, 26, 71, 62, 46],
# [63, 80, 77, 27, 51, 75, 43, 84, 87, 99],
# [11, 77, 90, 23, 71, 34, 33, 58, 56, 29],
# [ 4, 11, 19, 84, 57, 56, 32, 53, 43, 5]])
# 上下合并:垂直级联
np.vstack((n1, n2))
# array([[48, 89, 82, 88, 55],
# [63, 80, 77, 27, 51],
# [11, 77, 90, 23, 71],
# [ 4, 11, 19, 84, 57],
# [86, 26, 71, 62, 46],
# [75, 43, 84, 87, 99],
# [34, 33, 58, 56, 29],
# [56, 32, 53, 43, 5]])
5. 拆分
np.split / np.vsplit / np.hsplit
n = np.random.randint(0, 100, size=(6,4))
n
# array([[ 3, 90, 62, 89],
# [75, 7, 10, 76],
# [77, 94, 88, 59],
# [78, 66, 81, 83],
# [18, 88, 40, 81],
# [ 2, 38, 26, 21]])
# 垂直方向,平均切成3份
np.vsplit(n, 3)
# [array([[ 3, 90, 62, 89],
# [75, 7, 10, 76]]),
# array([[77, 94, 88, 59],
# [78, 66, 81, 83]]),
# array([[18, 88, 40, 81],
# [ 2, 38, 26, 21]])]
# 如果是数组
np.vsplit(n, (1,2,4))
# [array([[ 3, 90, 62, 89]]),
# array([[75, 7, 10, 76]]),
# array([[77, 94, 88, 59],
# [78, 66, 81, 83]]),
# array([[18, 88, 40, 81],
# [ 2, 38, 26, 21]])]
# 水平方向
np.hsplit(n, 2)
# [array([[97, 86],
# [16, 70],
# [26, 95],
# [ 6, 83],
# [97, 43],
# [96, 57]]),
# array([[88, 69],
# [60, 7],
# [32, 82],
# [24, 86],
# [62, 23],
# [43, 19]])]
# 通过axis来按照指定维度拆分
np.split(n, 2, axis=1)
# [array([[97, 86],
# [16, 70],
# [26, 95],
# [ 6, 83],
# [97, 43],
# [96, 57]]),
# array([[88, 69],
# [60, 7],
# [32, 82],
# [24, 86],
# [62, 23],
# [43, 19]])]
示例: 把猫拆分
cat.shape
# (456, 730, 3)
# 拆分
cat2 = np.split(cat, 2, axis=0)
cat2[0]
plt.imshow(cat2[0])
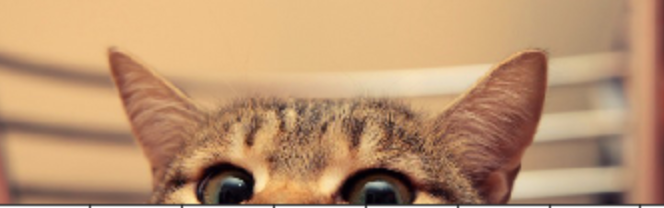
# 拆分成5分
cat2 = np.split(cat, 5, axis=1)
plt.imshow(cat2[2])
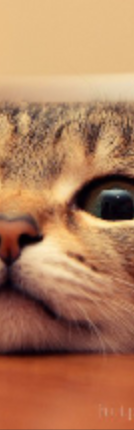
6. 拷贝/复制/副本
copy
# 赋值: 不使用copy
n1 = np.arange(10)
n2 = n1
n1[0] = 100
display(n1, n2)
# array([100, 1, 2, 3, 4, 5, 6, 7, 8, 9])
# array([100, 1, 2, 3, 4, 5, 6, 7, 8, 9])
# 拷贝: copy
n1 = np.arange(10)
n2 = n1.copy()
n1[0] = 100
display(n1, n2)
# array([100, 1, 2, 3, 4, 5, 6, 7, 8, 9])
# array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
7. 转置
# 转置
n = np.random.randint(0, 10, size=(3, 4))
n.T
# transpose改变数组维度
n = np.random.randint(0, 10, size=(3, 4, 5)) # shape(3, 4, 5)
np.transpose(n, axes=(2,0,1))