(给ImportNew加星标,提高Java技能)
作者:废物大师兄
www.cnblogs.com/cjsblog/p/9756978.html
1. 前言
1.1. 集成方式
Spring Boot中集成Elasticsearch有4种方式:
REST Client
Jest
Spring Data
Spring Data Elasticsearch Repositories
本文用后面两种方式来分别连接并操作Elasticsearch
1.2. 环境与配置
cluster.name: my-application
network.host: 192.168.1.134
http.port: 9200
/etc/security/limits.conf
cheng soft nofile 65536
cheng hard nofile 65536
/etc/sysctl.conf
vm.max_map_count=262144
1.3. 版本
Spring Boot 2.0.5默认的elasticsearch版本很低,这里我们用最新版本6.4.1
如果启动过程中出现
java.lang.NoClassDefFoundError: org/elasticsearch/common/transport/InetSocketTransportAddress
则说明,elasticsearch依赖的jar包版本不一致,统一改成6.4.1即可
另外,Spring Boot 2.0.5依赖的spring-data-elasticsearch版本是3.0.1,需要升级到3.1.0
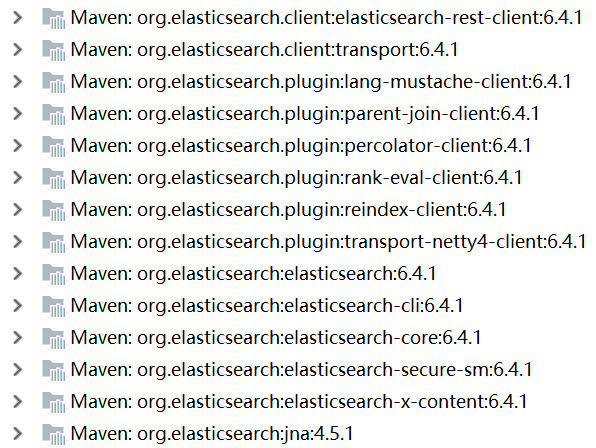
2. 依赖
"1.0" encoding="UTF-8"?>
"http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
4.0.0
com.cjs.example
cjs-elasticsearch-example
0.0.1-SNAPSHOT
jar
cjs-elasticsearch-example
org.springframework.boot
spring-boot-starter-parent
2.0.5.RELEASE
UTF-8
UTF-8
1.8
6.4.1
3.1.0.RELEASE
org.elasticsearch
elasticsearch
${elasticsearch.version}
org.elasticsearch.client
transport
${elasticsearch.version}
org.elasticsearch.client
elasticsearch-rest-client
${elasticsearch.version}
org.elasticsearch.plugin
transport-netty4-client
${elasticsearch.version}
org.springframework.data
spring-data-elasticsearch
${spring.data.elasticsearch.version}
org.springframework.boot
spring-boot-starter-data-elasticsearch
org.springframework.boot
spring-boot-starter-web
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-starter-test
test
org.springframework.boot
spring-boot-maven-plugin
3. application.properties
spring.data.elasticsearch.cluster-name=my-application
spring.data.elasticsearch.cluster-nodes=192.168.1.134:9300
也许,大家会疑惑,配置文件中明明写的端口是9200,为何这里配置文件中连接的时候写的端口是9300呢?
因为,配置9200是通过HTTP连接的端口,9300是TCP连接的端口
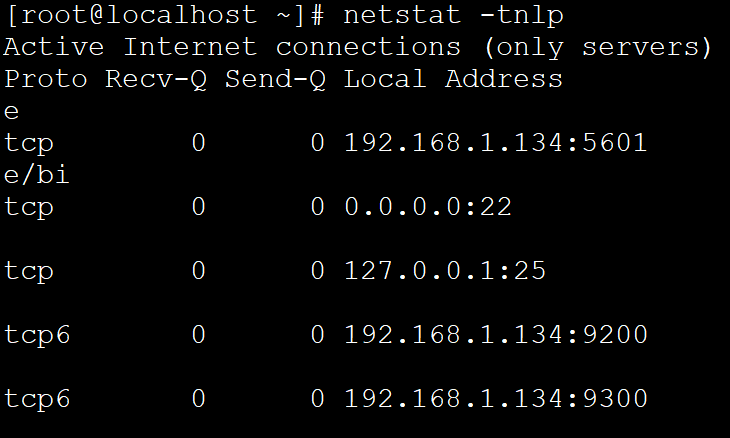
4. 操作
4.1. 使用Spring Data Elasticsearch Repositories操作Elasticsearch
首先,定义一个实体类
package com.cjs.example.entity;
import lombok.Data;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import java.io.Serializable;
@Data
@Document(indexName = "commodity")
public class Commodity implements Serializable {
@Id
private String skuId;
private String name;
private String category;
private Integer price;
private String brand;
private Integer stock;
}
这里定义了Commodity实例,表示商品。在Elasticsearch 6.X 版本中,不建议使用type,而且在7.X版本中将会彻底废弃type,所以此处我只指定了indexName,没有指定type。这里,一个Commodity代表一个商品,同时代表一条索引记录。
类比关系型数据库的话,Index相当于表,Document相当于记录
然后,需要自己定义一个接口,并继承ElasticsearchRepository
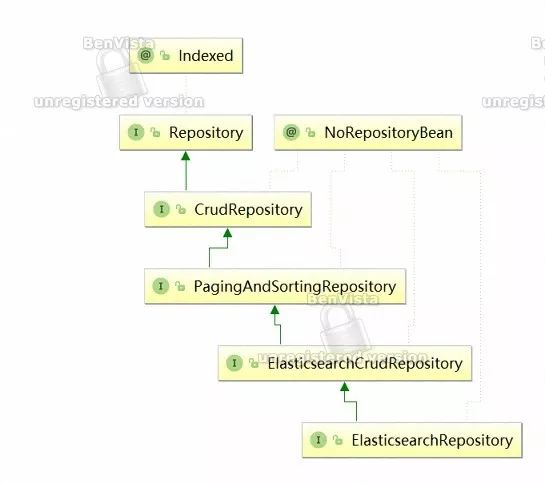
package com.cjs.example.dao;
import com.cjs.example.entity.Commodity;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface CommodityRepository extends ElasticsearchRepository {
}
这里的Repository相当于DAO,操作mysql还是elasticsearch都是一样的
接下来,定义service接口
package com.cjs.example.service;
import com.cjs.example.entity.Commodity;
import org.springframework.data.domain.Page;
import java.util.List;
public interface CommodityService {
long count();
Commodity save(Commodity commodity);
void delete(Commodity commodity);
Iterable getAll();
List getByName(String name);
Page pageQuery(Integer pageNo, Integer pageSize, String kw);
}
实现类
package com.cjs.example.service.impl;
import com.cjs.example.entity.Commodity;
import com.cjs.example.dao.CommodityRepository;
import com.cjs.example.service.CommodityService;
import org.elasticsearch.index.query.MatchQueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder;
import org.springframework.data.elasticsearch.core.query.SearchQuery;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
public class CommodityServiceImpl implements CommodityService {
@Autowired
private CommodityRepository commodityRepository;
@Override
public long count() {
return commodityRepository.count();
}
@Override
public Commodity save(Commodity commodity) {
return commodityRepository.save(commodity);
}
@Override
public void delete(Commodity commodity) {
commodityRepository.delete(commodity);
}
@Override
public Iterable getAll() {
return commodityRepository.findAll();
}
@Override
public List getByName(String name) {
List list = new ArrayList<>();
MatchQueryBuilder matchQueryBuilder = new MatchQueryBuilder("name", name);
Iterable iterable = commodityRepository.search(matchQueryBuilder);
iterable.forEach(e->list.add(e));
return list;
}
@Override
public Page pageQuery(Integer pageNo, Integer pageSize, String kw) {
SearchQuery searchQuery = new NativeSearchQueryBuilder()
.withQuery(QueryBuilders.matchPhraseQuery("name", kw))
.withPageable(PageRequest.of(pageNo, pageSize))
.build();
return commodityRepository.search(searchQuery);
}
}
在这个Service中演示了增删查改操作,还有分页查询
最后,写一个测试类测试其中的方法
package com.cjs.example;
import com.cjs.example.entity.Commodity;
import com.cjs.example.service.CommodityService;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.domain.Page;
import org.springframework.test.context.junit4.SpringRunner;
import java.util.List;
@RunWith(SpringRunner.class)
@SpringBootTest
public class CjsElasticsearchExampleApplicationTests {
@Autowired
private CommodityService commodityService;
@Test
public void contextLoads() {
System.out.println(commodityService.count());
}
@Test
public void testInsert() {
Commodity commodity = new Commodity();
commodity.setSkuId("1501009001");
commodity.setName("原味切片面包(10片装)");
commodity.setCategory("101");
commodity.setPrice(880);
commodity.setBrand("良品铺子");
commodityService.save(commodity);
commodity = new Commodity();
commodity.setSkuId("1501009002");
commodity.setName("原味切片面包(6片装)");
commodity.setCategory("101");
commodity.setPrice(680);
commodity.setBrand("良品铺子");
commodityService.save(commodity);
commodity = new Commodity();
commodity.setSkuId("1501009004");
commodity.setName("元气吐司850g");
commodity.setCategory("101");
commodity.setPrice(120);
commodity.setBrand("百草味");
commodityService.save(commodity);
}
@Test
public void testDelete() {
Commodity commodity = new Commodity();
commodity.setSkuId("1501009002");
commodityService.delete(commodity);
}
@Test
public void testGetAll() {
Iterable iterable = commodityService.getAll();
iterable.forEach(e->System.out.println(e.toString()));
}
@Test
public void testGetByName() {
List list = commodityService.getByName("面包");
System.out.println(list);
}
@Test
public void testPage() {
Page page = commodityService.pageQuery(0, 10, "切片");
System.out.println(page.getTotalPages());
System.out.println(page.getNumber());
System.out.println(page.getContent());
}
}
以上,便是使用Elasticsearch Repositories的方式
4.2. 使用ElasticsearchTemplate方式操作Elasticsearch
package com.cjs.example;
import com.cjs.example.entity.Commodity;
import org.elasticsearch.index.query.QueryBuilders;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.elasticsearch.core.ElasticsearchTemplate;
import org.springframework.data.elasticsearch.core.query.*;
import org.springframework.test.context.junit4.SpringRunner;
import java.util.List;
@RunWith(SpringRunner.class)
@SpringBootTest
public class ElasticsearchTemplateTest {
@Autowired
public ElasticsearchTemplate elasticsearchTemplate;
@Test
public void testInsert() {
Commodity commodity = new Commodity();
commodity.setSkuId("1501009005");
commodity.setName("葡萄吐司面包(10片装)");
commodity.setCategory("101");
commodity.setPrice(160);
commodity.setBrand("良品铺子");
IndexQuery indexQuery = new IndexQueryBuilder().withObject(commodity).build();
elasticsearchTemplate.index(indexQuery);
}
@Test
public void testQuery() {
SearchQuery searchQuery = new NativeSearchQueryBuilder()
.withQuery(QueryBuilders.matchQuery("name", "吐司"))
.build();
List list = elasticsearchTemplate.queryForList(searchQuery, Commodity.class);
System.out.println(list);
}
}
ElasticsearchTemplate是自动配置的
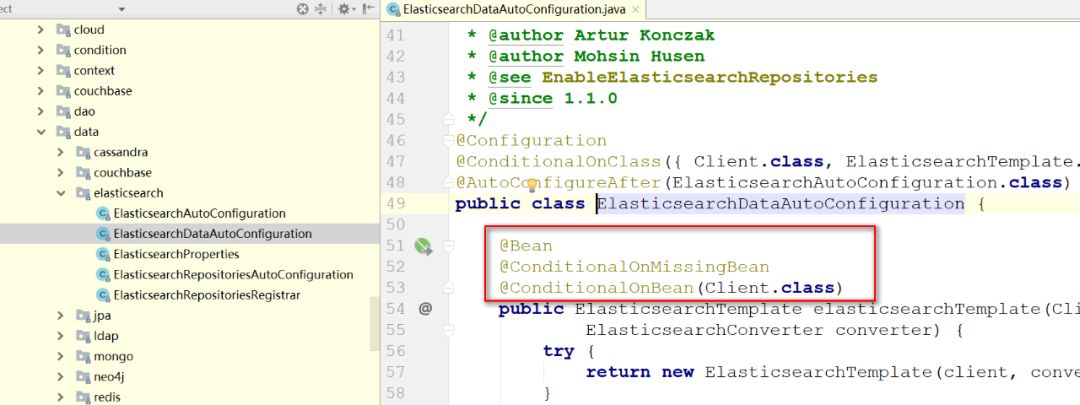
5. 演示
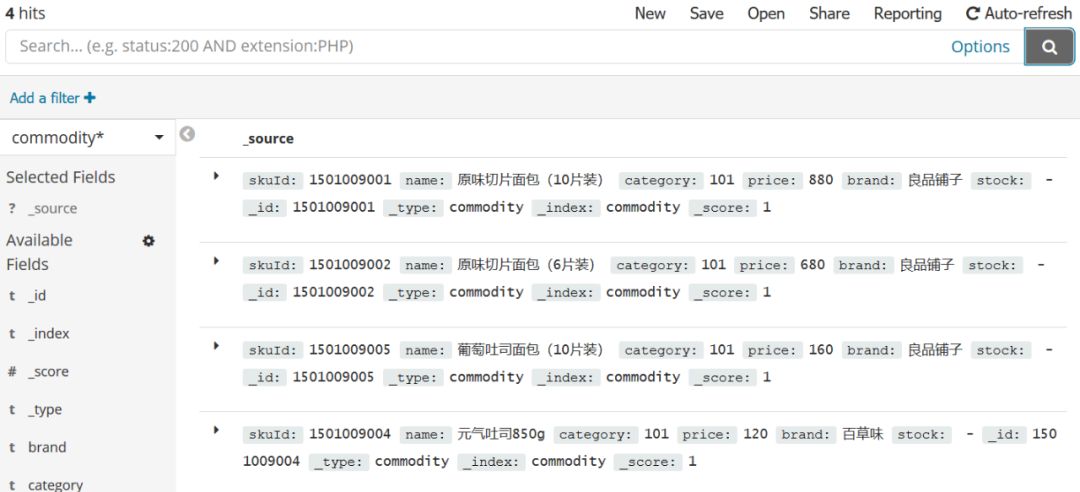
6. 工程结构
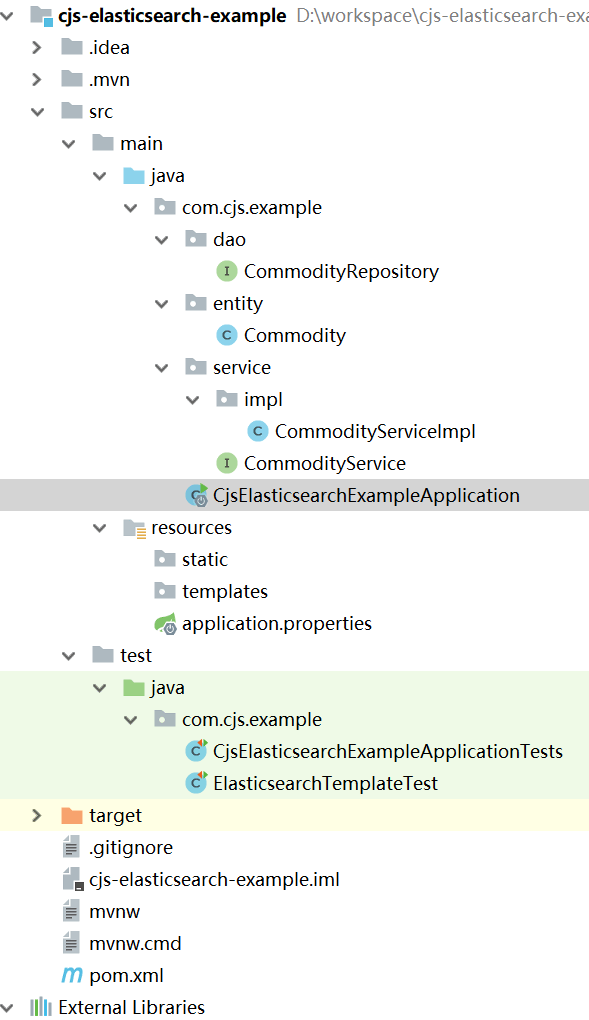
7. 参考

看完本文有收获?请转发分享给更多人
关注「ImportNew」,提升Java技能
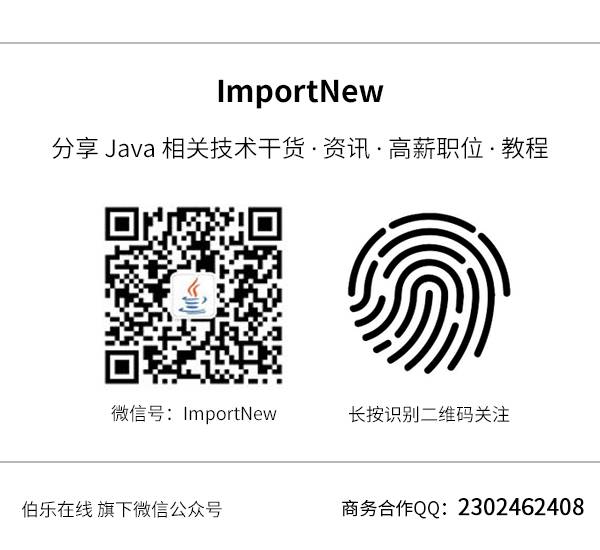
好文章,我在看❤️