区块链技术学习(微信号:Blockchain1024)翻译
原文:https://medium.com/@pemtajo/creating-a-blockchain-with-less-100-code-lines-7168849d687d
区块链的基本概念非常简单:一个分布式数据库,它维护一个不断增长的有序记录列表。
区块链是一个通常与比特币和/或以太坊相关的术语,但区块链不止于此,区块链是这些货币背后以及任何其他加密货币背后的技术。
区块链还有很多其他用途,例如游戏(CryptoKitties)或区块链+物联网(Internet of things),这只是这项技术的开始。
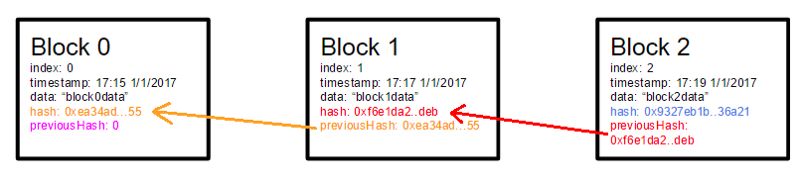
简单的区块链的概念
像所说的那样,区块链是一个块链,所以我们有第一类,区块。
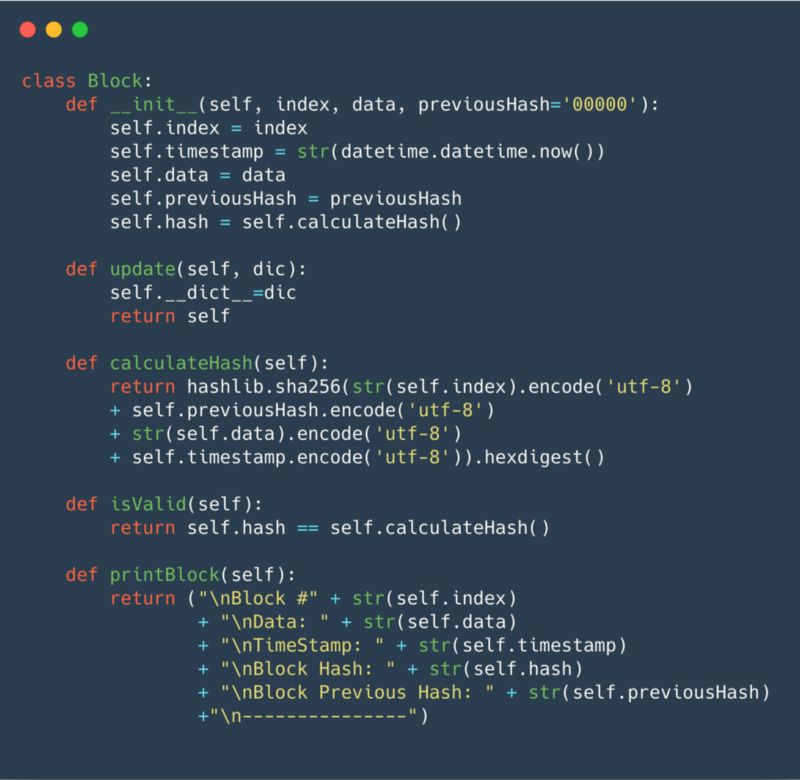
在这个阶段,我的块具有以下属性:
index - 块索引链中的位置
timestamp - 在区块链中添加块的日期和时间
data - 数据值,换句话说,就是你想保存的东西
previous hash - 块索引-1的哈希值
hash - 块的哈希值
如果你不知道什么是哈希,可以看看之前的文章《据说,80%的人都搞不懂哈希算法》
你会在图片中看到一些有趣的东西,在这里我会解释一下:
更多的OOP函数isValid是针对每个块的,如果每个块响应有效,则该函数是有效的。
构造函数定义了块中的所有东西。
函数“update”是在从文件读取时更新dict,这是为了将来保存数据。
计算以前保存的文件的哈希值,总是转换为相同的编码,因为不同的编码具有不同的字符,而不同的字符产生不同的哈希值。
所以,这是一个有效的链,如果块被改变了,当前的块就会知道,并使自己无效,如果之前的任何块被改变了,这个链就会知道,并且所有链将无效。这就是使区块链中的数据保存不可变的概念。
所以看看我们接下来要说的区块链,它看起来像:
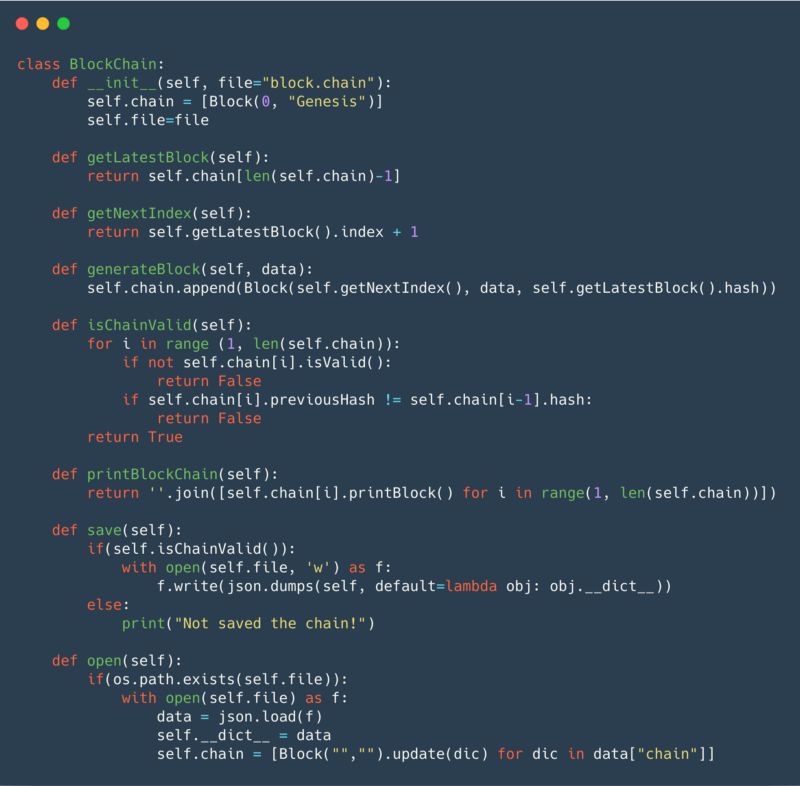
因此,区块链类创建块并在链中查找任何问题,这个类负责保存在一个简单的JSON文件中并从它那里读取数据。我们的第一个版本区块链已经准备好了。
所有代码都在下面,您可以执行并查看输出。
import json
import os
import hashlib
import datetime
class Block:
def __init__(self, index, data, previousHash='00000'):
self.index = index
self.timestamp = str(datetime.datetime.now())
self.data = data
self.previousHash = previousHash
self.hash = self.calculateHash()
def update(self, dic):
self.__dict__=dic
return self
def calculateHash(self):
return hashlib.sha256(str(self.index).encode('utf-8')
+ self.previousHash.encode('utf-8')
+ str(self.data).encode('utf-8')
+ self.timestamp.encode('utf-8')).hexdigest()
def isValid(self):
return self.hash == self.calculateHash()
def printBlock(self):
return ("\nBlock #" + str(self.index)
+ "\nData: " + str(self.data)
+ "\nTimeStamp: " + str(self.timestamp)
+ "\nBlock Hash: " + str(self.hash)
+ "\nBlock Previous Hash: " + str(self.previousHash)
+"\n---------------")
class BlockChain:
def __init__(self, file="block.chain"):
self.chain = [Block(0, "Genesis")]
self.file=file
def getLatestBlock(self):
return self.chain[len(self.chain)-1]
def getNextIndex(self):
return self.getLatestBlock().index + 1
def generateBlock
(self, data):
self.chain.append(Block(self.getNextIndex(), data, self.getLatestBlock().hash))
def isChainValid(self):
for i in range (1, len(self.chain)):
if not self.chain[i].isValid():
return False
if self.chain[i].previousHash != self.chain[i-1].hash:
return False
return True
def printBlockChain(self):
return ''.join([self.chain[i].printBlock() for i in range(1, len(self.chain))])
def save(self):
if(self.isChainValid()):
with open(self.file, 'w') as f:
f.write(json.dumps(self, default=lambda obj: obj.__dict__))
else:
print("Not saved the chain!")
def open(self):
if
(os.path.exists(self.file)):
with open(self.file) as f:
data = json.load(f)
self.__dict__ = data
self.chain = [Block("","").update(dic) for dic in data["chain"]]
def main():
blockchain = BlockChain()
blockchain.generateBlock("Hello World!")
blockchain.generateBlock(3)
blockchain.generateBlock({"account": 123123, "mount": 100})
print(blockchain.printBlockChain())
print ("Chain valid? " + str(blockchain.isChainValid()))
blockchain.save()
blockchain.chain[1].data = "Hello Darkness my old friend!"
print(blockchain.printBlockChain())
print ("Chain valid? " + str(blockchain.isChainValid()))
blockchain.save()
test = BlockChain()
test.open()
print(test.printBlockChain())
print ("Chain valid? " + str(test.isChainValid()))
test.save()
if __name__ == '__main__':
main()
在这个版本的区块链中,我们没有实现工作证明,首先创建了区块链,并保证了链的集成。这将是我的下一步。
创建了一个项目,你可以在GitHub上跟进,如果你有兴趣,就跟着我,我会写一些关于整个过程的文章。
GitHub地址:https://github.com/pemtajo/blockchain
PS:我不是区块链方面的专家,所以如果有任何问题,比如代码修复,或提示,欢迎大家在下面评论,也会帮助一些人。或者你也可以在LinkedIn上私下和我聊天。
LinkedIn地址:https://www.linkedin.com/in/pedromaraujo/
Pedro MatiasdeAraújo
Fornax Tecnologia的软件开发人员,有3年以上的开发经验,解决问题和优化算法的经验。
喜欢做和学习不同寻常的事,比如练习美式足球六年,直到创建了一个关于密码学的博客(I believe that Blockchain will change the world),并开始学习法语和日语。
●编号176,输入编号直达本文
●输入m获取文章目录
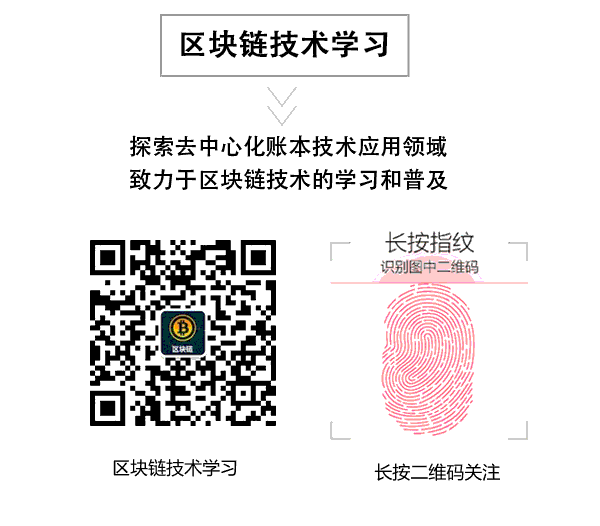