转载:
https://www.cnblogs.com/connect/p/python-wechat-iciba.html
实验环境
阿里云Linux服务器
Python环境
爱词霸每日一句API介绍
调用地址:http://open.iciba.com/dsapi/
请求方式:GET
请求参数:

返回类型:JSON
JSON字段解释:
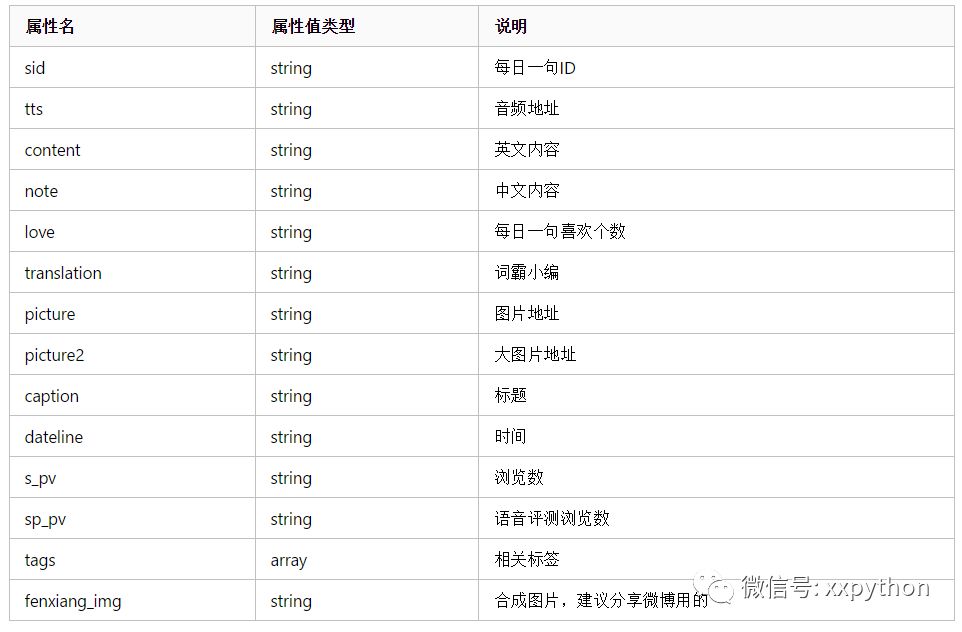
正常返回示例:
{
"sid": "3080",
"tts": "http://news.iciba.com/admin/tts/2018-08-01-day.mp3",
"content": "No matter how hard we try to be mature, we will always be a kid when we all get hurt and cry. ",
"note": "不管多努力蜕变成熟,一旦受伤哭泣时,我们还是像个孩子。",
"love": "1966",
"translation": "小编的话:这句话出自小说《彼得·潘》。岁月永远年轻,我们慢慢老去。不管你如何蜕变,最后你会发现:童心未泯,是一件值得骄傲的事情。长大有时很简单,但凡事都能抱着一颗童心去快乐享受却未必容易。",
"picture": "http://cdn.iciba.com/news/word/20180801.jpg",
"picture2": "http://cdn.iciba.com/news/word/big_20180801b.jpg",
"caption": "词霸每日一句",
"dateline": "2018-08-01",
"s_pv": "0",
"sp_pv": "0",
"tags": [
{
"id": null,
"name": null
}
],
"fenxiang_img": "http://cdn.iciba.com/web/news/longweibo/imag/2018-08-01.jpg"
}
请求示例:
Python2请求示例
import json
import urllib2
def get_iciba_everyday():
url = 'http://open.iciba.com/dsapi/'
request = urllib2.Request(url)
response = urllib2.urlopen(request)
json_data = response.read()
data = json.loads(json_data)
return data
print get_iciba_everybody()
Python3请求示例
import json
import requests
def get_iciba_everyday():
url = 'http://open.iciba.com/dsapi/'
r = requests.get(url)
return json.loads(r.text)
print(get_iciba_everyday())
PHP请求示例
function https_request($url, $data = null){
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_HEADER, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 0);
if (!empty($data)) {
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
}
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$output = curl_exec($curl);
curl_close($curl);
return $output;
}
function get_iciba_everyday(){
$url = 'http://open.iciba.com/dsapi/'
$result = https_request($url);
$data = json_decode($result);
return $data;
}
echo get_iciba_everyday();
登录微信公众平台接口测试账号
扫描登录公众平台测试号
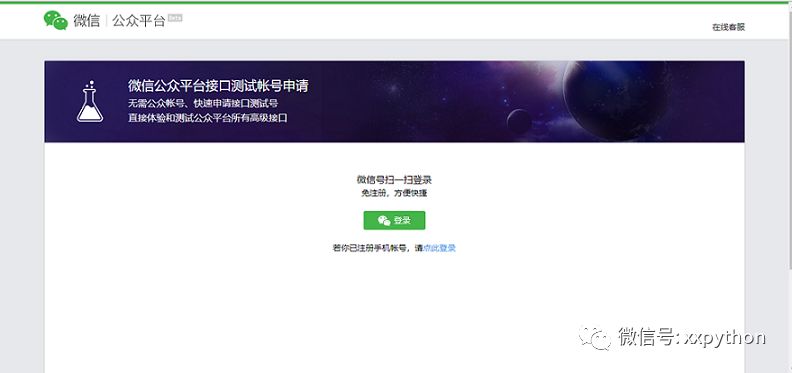
手机上确认登录
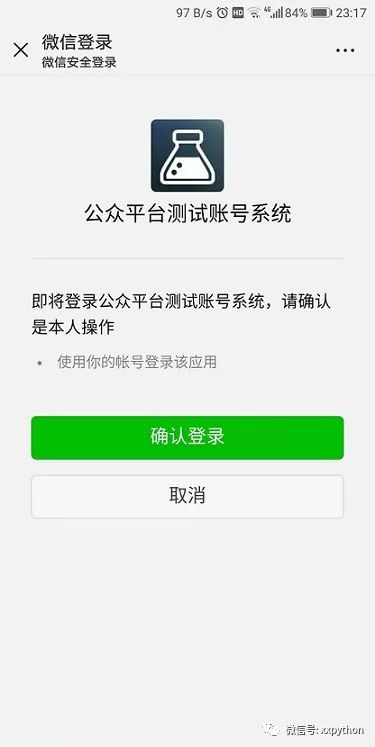
找到新增测试模板
,添加模板消息

填写模板标题每日一句
,填写如下模板内容
{{content.DATA}}
{{note.DATA}}
{{translation.DATA}}
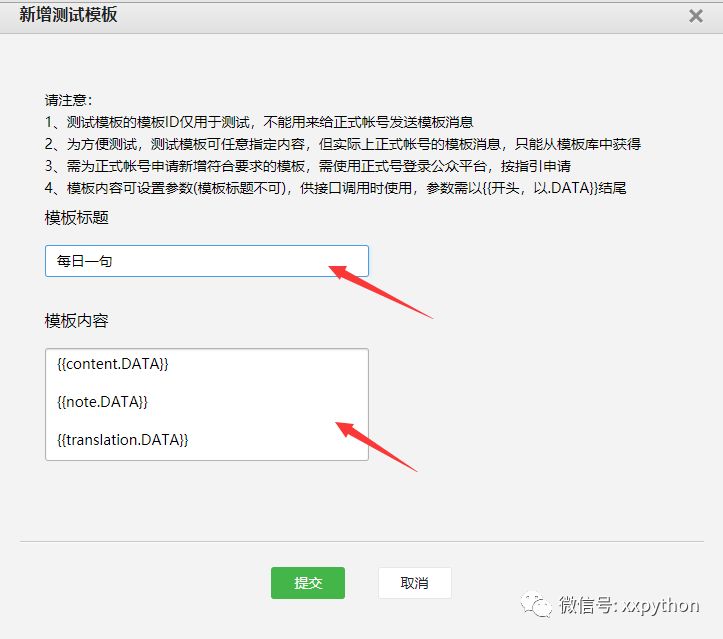
提交保存之后,记住该模板ID
,一会儿会用到
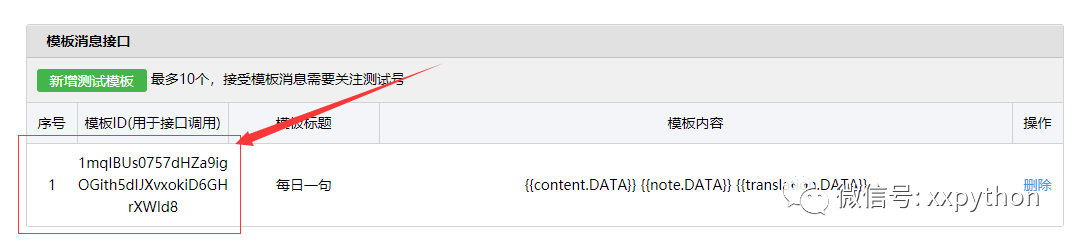
找到测试号信息
,记住appid
和appsecret
,一会儿会用到

找到测试号二维码
。手机扫描此二维码,关注之后,你的昵称会出现在右侧列表里,记住该微信号,一会儿会用到(注:此微信号非你真实的微信号)
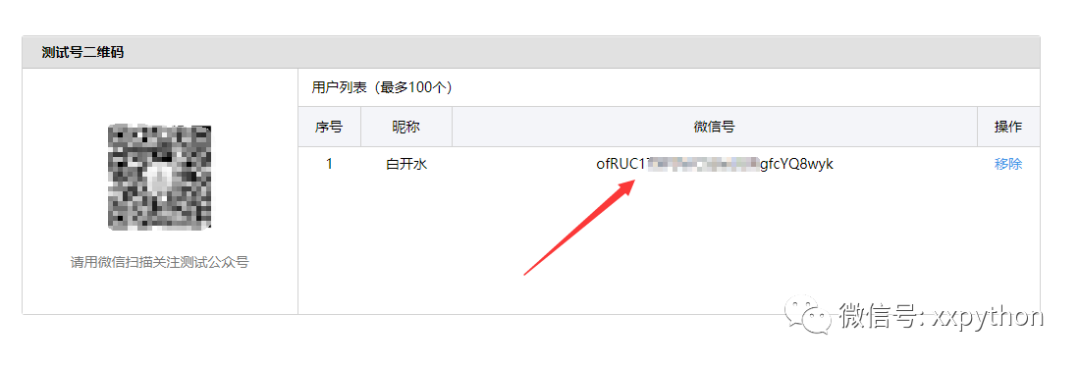
发送微信模板消息的程序
本程序您只需要修改4个地方即可,请看注释
Python2实现
import json
import urllib2
class iciba:
def __init__(self, wechat_config):
self.appid = wechat_config['appid']
self.appsecret = wechat_config['appsecret']
self.template_id = wechat_config['template_id']
self.access_token = ''
def get_access_token(self, appid, appsecret):
url = 'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=%s&secret=%s' % (appid, appsecret)
request = urllib2.Request(url)
response = urllib2.urlopen(request)
json_data = response.read()
data = json.loads(json_data)
access_token = data['access_token']
self.access_token = access_token
return self.access_token
def get_user_list(self):
if self.access_token == '':
self.get_access_token(self.appid, self.appsecret)
access_token = self.access_token
url = 'https://api.weixin.qq.com/cgi-bin/user/get?access_token=%s&next_openid=' % str(access_token)
request = urllib2.Request(url)
response = urllib2.urlopen(request)
result = response.read()
return
json.loads(result)
def send_msg(self, openid, template_id, iciba_everyday):
msg = {
'touser': openid,
'template_id': template_id,
'url': iciba_everyday['fenxiang_img'],
'data': {
'content': {
'value': iciba_everyday['content'],
'color': '#0000CD'
},
'note': {
'value': iciba_everyday['note'],
},
'translation': {
'value': iciba_everyday['translation'],
}
}
}
json_data = json.dumps(msg)
if self.access_token == '':
self.get_access_token(self.appid, self.appsecret)
access_token = self.access_token
url = 'https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=%s' % str(access_token)
request = urllib2.Request(url, data=json_data)
response = urllib2.urlopen(request)
result = response.read()
return json.loads(result)
def get_iciba_everyday(self):
url = 'http://open.iciba.com/dsapi/'
request = urllib2.Request(url)
response = urllib2.urlopen(request)
json_data = response.read()
data = json.loads(json_data)
return data
def send_everyday_words(self, openids):
everyday_words = self.get_iciba_everyday()
for openid in openids:
result = self.send_msg(openid, self.template_id, everyday_words)
if result['errcode'] == 0:
print ' [INFO] send to %s is success' % openid
else:
print ' [ERROR] send to %s is error' % openid
def run(self, openids=[]):
if openids == []:
result = self.get_user_list()
openids = result['data']['openid']
self.send_everyday_words(openids)
if __name__ == '__main__':
wechat_config = {
'appid': 'xxxxx',
'appsecret': 'xxxxx',
'template_id': 'xxxxx'
}
openids = [
'xxxxx',
]
icb = iciba(wechat_config)
icb.run()
Python3实现
import json
import requests
class iciba:
def __init__(self, wechat_config):
self.appid = wechat_config['appid']
self.appsecret = wechat_config['appsecret']
self.template_id = wechat_config['template_id']
self.access_token = ''
def get_access_token(self, appid, appsecret):
url = 'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=%s&secret=%s' % (str(appid), str(appsecret))
r = requests.get(url)
data = json.loads(r.text)
access_token = data['access_token']
self.access_token = access_token
return self.access_token
def get_user_list(self):
if self.access_token == '':
self.get_access_token(self.appid, self.appsecret)
access_token = self.access_token
url = 'https://api.weixin.qq.com/cgi-bin/user/get?access_token=%s&next_openid=' % str(access_token)
r = requests.get(url)
return json.loads(r.text)
def send_msg(self, openid, template_id, iciba_everyday):
msg = {
'touser': openid,
'template_id': template_id,
'url': iciba_everyday['fenxiang_img'],
'data': {
'content': {
'value': iciba_everyday['content'],
'color': '#0000CD'
},
'note': {
'value': iciba_everyday['note'],
},
'translation': {
'value': iciba_everyday['translation'],
}
}
}
json_data = json.dumps(msg)
if self.access_token == '':
self.get_access_token(self.appid, self.appsecret)
access_token = self.access_token
url = 'https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=%s' % str(access_token)
r = requests.post(url, json_data)
return json.loads(r.text)
def get_iciba_everyday
(self):
url = 'http://open.iciba.com/dsapi/'
r = requests.get(url)
return json.loads(r.text)
def send_everyday_words(self, openids):
everyday_words = self.get_iciba_everyday()
for openid in openids:
result = self.send_msg(openid, self.template_id, everyday_words)
if result['errcode'] == 0:
print (' [INFO] send to %s is success' % openid)
else:
print (' [ERROR] send to %s is error' % openid)
def run(self, openids=[]):
if openids == []:
result = self.get_user_list()
openids = result['data']['openid']
self.send_everyday_words(openids)
if __name__ == '__main__':
wechat_config = {
'appid': 'xxxxx',
'appsecret': 'xxxxx',
'template_id': 'xxxxx'
}
openids = [
'xxxxx',
]
icb = iciba(wechat_config)
icb.run()
测试程序
在Linux上执行程序
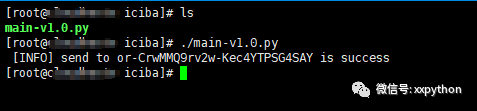
在手机上查看,已经收到了每日一句的消息
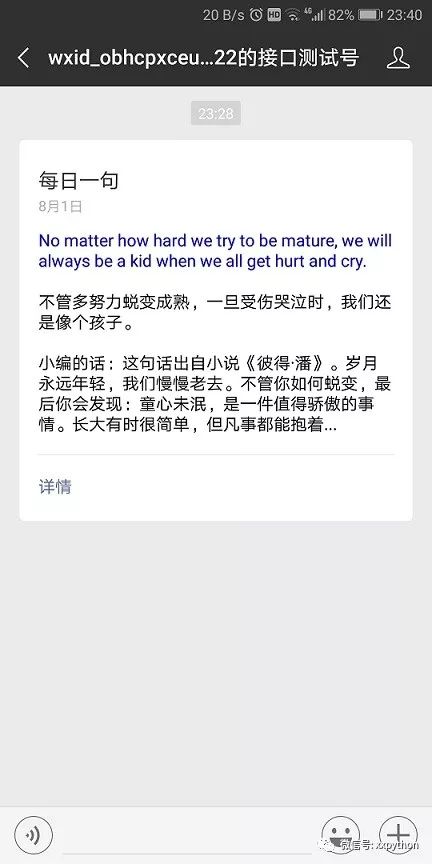
部署程序
在Linux上设置定时任务
crontab -e
添加如下内容
0 6 * * * python /root/python/iciba/main-v1.0.py
注:以上内容的含义是,在每天6:00
的时候,执行这个Python程序
查看定时任务是否设置成功
crontab -l
至此,程序部署完成,请您明天6:00
查收吧!
效果图如下
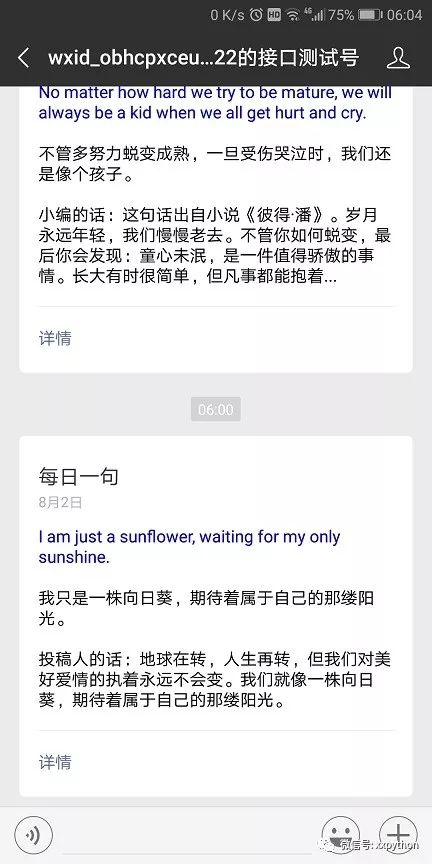